Overview
Vision Statement
We will provide a stream-lined and simple-to-use application for managing and playing games of Assassins. Players can easily start and join games, as well as track their statistics and manage a friends list. Game creators will be able to deal with disputes during the games and roll back to previous game states as necessary. Players report their kills and are informed of the changes to the game state (deaths, new targets).
Assassins is a game in which players are assigned specific targets and, through some pre-defined method, must "kill" their targets (thus removing them from the game). The last player standing wins.
Features List
Key Features
- Players can...
- create an account on a server and log-in via a web interface (all of the actions of players are done through this web interface)
- start games of Assassins and invite other players on that server
- receive and respond to these invites and join these games
- report their kills to the server
- The server will handle the maintaining of the kill loops and updating all players to kills and changes in the game
- Game creators can...
- customize the settings of their game at creation
- optionally join (as players) the games they create
- kick players mid-game if necessary
- appoint administrators for games
- Administrators arbitrate disputes within a game
- Server operators can...
- configure custom settings on their server
- import and export statistics to and from their servers
Rules
- Someone starts a game of assassins and invites people they want to play
- Each player is assigned a target according to some assignment scheme, usually a randomly-arranged cyclic graph.
- At a certain start date, the game begins. All players are thusly informed of their targets.
- Play involves players attempting to "kill" their target. Killing can constitute any number of actions, but in general, killing consists of a player tapping or poking his or her target with a plastic spoon.
- Players are only allowed to kill their specified target (in some games, a "wanted" list exists, and all players on the list can be legally killed by all other players). Killing a player you are not supposed to is invalid and often results in a penalty of some kind.
- Upon killing a player, some central authority is notified (in traditional games this is whoever set up the game, who is generally not participating. In our program, this authority would be the server) by the assassin (in most games, the killed target must agree that they were killed, and in some games the killed target must give their assassin their plastic spoon)
- The central authority removes the dead player from the game and changes the kill order accordingly. In most games, this means that whoever the newly dead player had as their target becomes the target of the assassin
- The central authority notifies the assassin of their new target (in some games, the central authority notifies all players that a kill occurred)
- When there is only one player remaining alive, the game is over, and that remaining player is the winner.
Optional Rules
- A set stopping time, at which point the game ends and the player with the most kills becomes the winner
- A time limit for kills and an accompanying penalty, some games require that all assassins kill someone with a certain frequency or else be moved to a "wanted" list.
- The option for a target to be able to defend itself by killing its attacker, for example : Let us assume that Bob is Annie's target. Bob is sitting in the corner of the library. Annie not-so-subtly jumps in front of Bob, wildly yielding her spoon. Bob is cornered, and cannot run away. Instead of accepting his fate as a victim, he can now pull out the spoon in his pocket and "kill" Annie before Annie kills him. This kill will be treated as valid, although Annie was not Bob's target.
- There are many more "eccentric" rules that are added sometimes in games, but many are outside the scope of this project. If we have time, it may be possible to implement the following:
- Revival of dead players under certain circumstances
- Perks for fast or back-to-back kills ("killstreaks")
Extended Features
- Mobile clients, specifically on the Android platform, that would allow players to connect to a server and log-in, and afford them all the actions available to them through the web interface with enhanced usability for mobile devices deaths across all games and present those statistics on their
- Track statistics to a player's account and display a history of kills and deaths, and display interesting representations of the data on their player profiles, to be viewed by other players
- Alternative kill graph layouts to enable team play, as well as a possible "free for all" mode where everyone can attack everyone else
- Extension of configuration options through some form of custom scripting
- Extremely customizable games, with possible extension to any kind of group game with a similar structure to Assassins, such as Humans vs. Zombies or other forms of live-action games
- The ability to set certain times, on a per-game basis, that are "safe hours," during which no kills can occur
GUI Sketches
Architecture
We will be creating a deployable server package that can easily be run on any computer. We will be deploying our instance to a virtual machine hosted by the JHU ACM.
Each server is standalone. Clients can connect to the server through a web interface (or through a mobile Android client, if we have time to implement that) and access all user and game data. The server handles all of our use-cases involving player interaction.
A slightly more detailed view of our architecture:
We will use servlets to communicate between the Web application and the Tomcat server and the Tomcat server will use JSP to create the web interface that our users will connect to in order to play the game.
We will be using Spring to manage the interactions between the model and the view controller in our project.
We will be using Hibernate to interface with a PostgreSQL database to store player and game data.
We have our code divided into two parts, one for front end work and one for backend. The front end package consists entirely of code for creating the GUI (the View and Controller) while the backend package contains all the code for modelling the actual game. (The latter is what we have described in our class diagram).
Additionally, there may be additional client packages that can access this server from Android devices in which case we will be using a JSON interface. The primary way to interact with the server will be via a web interface. (The most likely additional client to be implemented will be an Android interface, as noted).
Use-Cases
Deploying a Server
Primary Actor: Server Operator
Secondary Actors: Server
Goal: Create a server
Pre-Condition: Someone wants to host a server
Post-Condition: Server is running now
Main Path:
- Server Operator creates a configuration file (or uses the default).
- Server Operator sets up their system to allow necessary connections.
- Server Operator launches the server.
Creating a Game
Primary Actor: Game Creator (a user)
Secondary Actors: Server, Players
Pre-Condition: Game creator has an account on the desired server, Server exists
Post-Condition: A new game has been added to the Server
Main Path:
- Game Creator logs into the Server of their choice via its web interface
- Game Creator creates a new game via the interface
- Game Creator invites Players on the Server
- The Server informs all Players of their invitations
Joining a Game
Primary Actor: Player
Secondary Actors: Server
Pre-Condition: Player has been invited to a game
Post-Condition: Player is now part of the game they were invited to
Main Path:
- Player logs into the Server via the web interface
- Player sees their invitations
- Player accepts an invitation
- Server adds the Player to that game and notifies the Game Creator
Reporting a Kill
Primary Actor: Player
Secondary Actors: Server, second Player (Victim), Administrators
Pre-Condition: The Victim is killed by the Player
Main Path:
- The Player logs into the web interface and reports their kill
- The Victim logs into the web interface and confirms their death
- The Server updates the target graphs and informs the Player of their new target
- The Server updates the data for all involved Players
Extensions:
- From step 2, if the Victim disputes the kill, an Administrator is asked to arbitrate the dispute and approve or deny the kill
- From step 3, if the game is configured in such a way, the Server may update all players that a kill took place
- From step 3, if this is the last kill then the Server will update all players that the game is now over at that the Player has won.
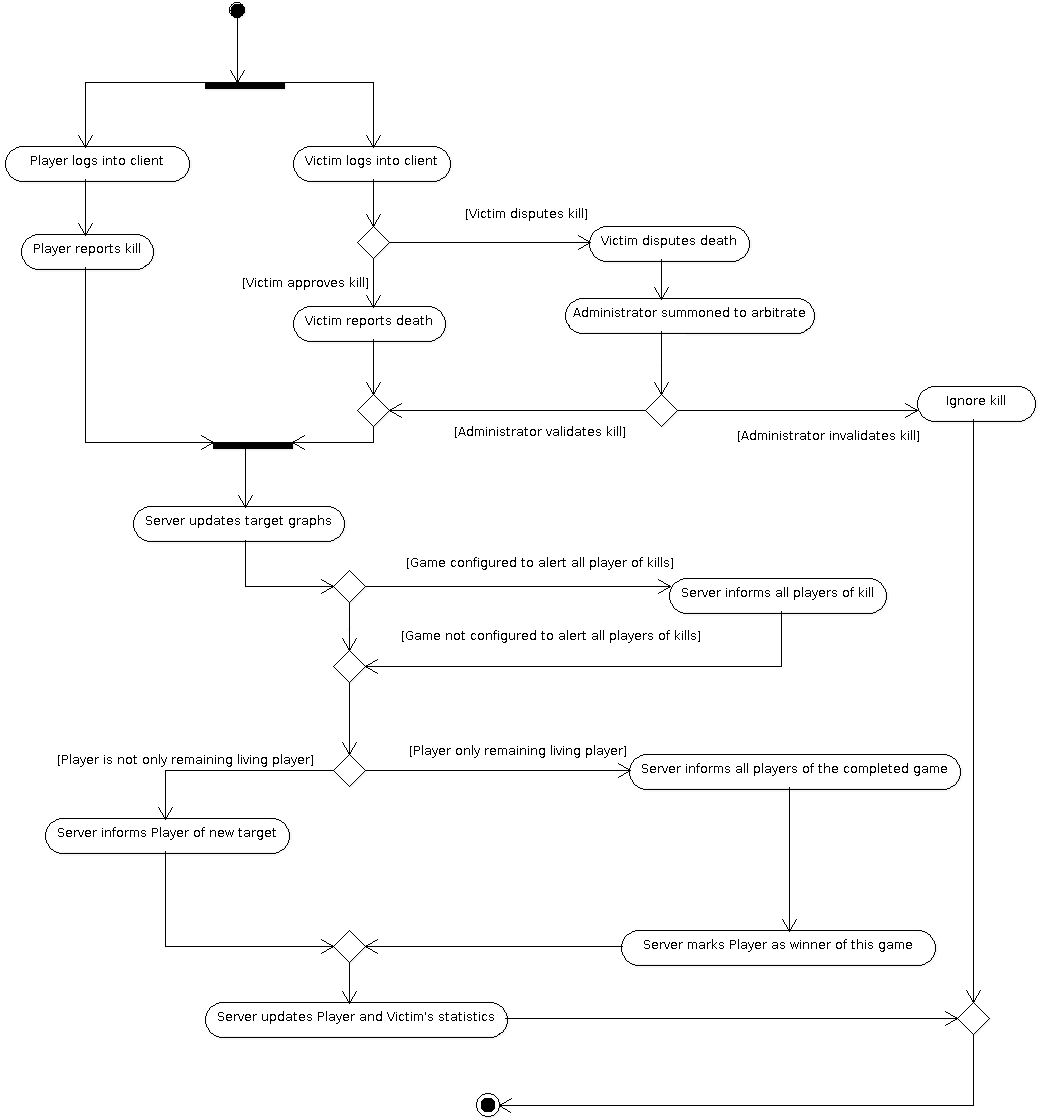
Creating an Account
Primary Actor: Player
Secondary Actors: Server, Server Operator
Main Path:
- A person opens the web interface for the server they want to play on
- This person enters the create an account dialog
- This person enters their information and account preferences
- The Server creates a new account an maintains it
Extensions:
- From step 3, the Server Operator can require that all accounts be approved by them. In this case, the Server notifies the Server Operator who can choose to approve or deny the account creation
Invalidating a Kill
Primary Actor: Administrator
Secondary Actor: Server, Killer, Victim
Main Path:
- The Administrator receives a notices that a kill was disputed by the Victim
- The Administrator, possibly outside the game, contacts the Killer and Victim
- The Administrator weighs evidence and decides whether the kill is valid
- The Administrator invalidates the kill in the game's interface
- The Server does not change the game state and clears the notices regarding the dispute
Validating a Kill
Primary Actor: Administrator
Secondary Actor: Killer, Victim
Main Path:
- The Administrator receives a notices that a kill was disputed by the Victim
- The Administrator, possibly outside the game, contacts the Killer and Victim
- The Administrator weighs evidence and decides whether the kill is valid
- The Administrator validates the kill in the game's interface
- The Server updates the game state in the same way it would as if the kill had never been disputed
Kicking a Player
Primary Actor: Administrator
Secondary Actor: Server, Player
Main Path:
- The Administrator goes to the interface for the game they are running
- The Administrator clicks the "Kick Player" button corresponding to the player that is to be kicked
- The Server removes the Player from the game and alerts them of this fact
- The Server updates the game as if the Player had been killed
Class Design Proposal
Here are the Javadocs for our classes.
Class Diagram
Class Design Diagram
Resources
- Java standard library
- Android SDK
- JUnit Testing Framework
- Java application server (tomcat)
- JSP/JSTL
- Servlets
- Data persistance (Hibernate)
- Data transfer (standard Java JSON parser)
- Spring